WooCommerce Product Sync Pro has hooks and filters which can be used to modify it’s behavior. For example, you can do the following:
- Change product prices so that the other site has different prices
- Prevent syncing of a certain product
- Map one field to another
You can try the examples below with Code Snippets or add them to your theme’s functions.php file.
wps_product_fields
The filter is used to modify product data before it’s sent to the receiving site.
Example: increase product price by 10 %
<?php
add_filter( 'wps_product_fields', function( $product_fields, $product, $site ) {
$site_url = 'example.com'; // change to your site URL, no https prefix is needed
if ( strpos( strtolower( $site->get_url() ), $site_url ) !== false ) {
$product_fields['regular_price'] *= 1.1; // increase by 10 %
}
return $product_fields;
}, 10, 3 );
Please note that this snippet can only be used in one-way syncing. If it’s applied to two-way syncing, price would increase by 10 % every time the product is updated.
Example 2: set meta data
<?php
add_filter( 'wps_product_fields', function( $product_fields, $product, $site ) {
$site_url = 'example.com'; // change to your site URL, no https prefix is needed
$meta_value = 'my_value';
if ( strpos( strtolower( $site->get_url() ), $site_url ) !== false ) {
$product_fields['meta_data']['_my_meta_field'] = [
'key' => '_my_meta_field',
'value' => base64_encode( serialize( $meta_value ) ),
];
}
return $product_fields;
}, 10, 3 );
woocommerce_rest_pre_insert_product_object
The filter is used to modify product data before it’s saved on the receiving website.
Example: save additional meta data
<?php
add_filter( 'woocommerce_rest_pre_insert_product_object', function( $product, $request, $creating ) {
update_post_meta( $product->get_id(), '_is_synced', true );
return $product;
}, 10, 3 );
wps_should_sync_to_site
The filter is used to determine whether or not the product should be synced to a particular site. Applies when a product has been edited and when running the Push All tool.
Example: only sync if the product is published and the site ID is 50
<?php
add_filter( 'wps_should_sync_to_site', function( $should_sync, $product_id, $variation_id, $site_id ) {
// Note that if the product is variation, $product_id will be the parent ID
// and $variation_id the original product ID of the variation
$product = wc_get_product( $product_id );
if ( $product && $site_id == 50 && $product->get_status( 'edit' ) == 'publish' ) {
return true;
}
return false;
}, 10, 4 );
Tip! You can find the site ID by hovering over the site in the settings:
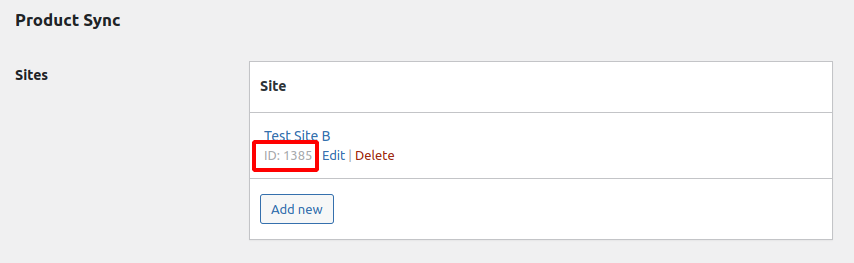
woo_product_sync_should_sync
The filter is used to determine whether or not the product should be synced when a product has been edited. Note! This doesn’t apply to Push All tool.
Example: only sync if the product has the category with the slug acme
<?php
add_filter( 'woo_product_sync_should_sync', function( $should_sync, $product ) {
$slug = 'acme';
// Get product categories
$cat_slugs = wp_get_post_terms( $product->get_id(), 'product_cat', [
'hide_empty' => false,
'fields' => 'slugs',
] );
// Stop syncing if the product doesn't have the category with the slug "acme"
if ( ! in_array( $slug, $cat_slugs, true ) ) {
return false;
}
return $should_sync;
}, 10, 2 );
Need anything else?
If the hook you were looking for was not listed, please let us know and our developers are happy to check if it’s possible to add.